I was recently building a contact form for a client using the popular Contact Form 7 WordPress plugin.
Like many forms, this form I was building had a drop down and one of the choices on the drop down was “Other”. The client wanted to display a text box if the user selected “Other”.
This sounds like it would be a common request right? It is. However, Contact Form 7 does not natively support conditional fields yet (ver 4.8.1).
There is a sweet add-on plugin for Contact Form 7 that will do that. And if you search online, there are many tutorials like this that in my opinion is an overkill for what could be done with some simple JavaScript.
Sure, if you have too many conditional fields, go for the add-on plugin. It will be cleaner and easier to manage. But for a lot of users with one or two conditional fields per form, the following should work just fine.
Inline JavaScript On Contact Form 7
If you add JavaScript into the Contact Form 7 form builder, it will run on the front-end on the page where the form is displayed. How neat!
Be warned! Do not add empty lines in the script or the form will add paragraph <p> and break the script. Other than that, inline js works just fine.
Conditionally Display Text Field When “Other” Is Selected In A Drop Down
For this example, I will build a simple contact form that accepts:
- Name
- Favorite Color (Pink, Red, Purple or Other)
- Text Field to specify the favorite color
Create a new form in Contact Form 7 and start by adding all the elements you want to be displayed on the form. Remember to specify a unique CSS ID for the Favorite Color drop down.
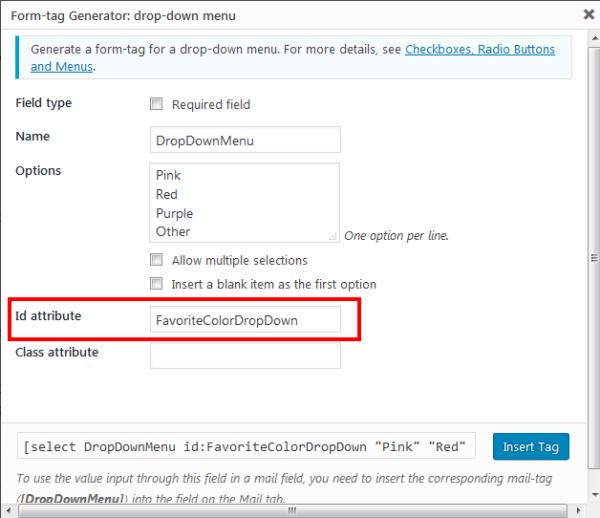
Drop Down Builder With Unique ID
Add the text field and a label and specify a unique CSS ID to the label. The form builder will look like this right now.
<label> Your Name (required)
[text* your-name] </label>
<label> Your Email (required)
[email* your-email] </label>
<label> Your Favorite Color
[select drop-down-menu id:FavoriteColorDropDown "Pink" "Red" "Purple" "Other"] </label>
<label id="EnterFavoriteColorLabel"> Please Specify Your Favorite Color
[text favorite-color] </label>
[submit "Send"]
Note that the label for favorite-color text field has the CSS ID ‘EnterFavoriteColorLabel‘.
Here is the JavaScript to display the text field when the option ‘Other‘ is selected in the dropdown with the ID ‘FavoriteColorDropDown‘.
The inline comments explain the script pretty well.
<script language="javascript" type="text/javascript">
// Hide the favorite-color text field by default
document.getElementById("EnterFavoriteColorLabel").style.display = 'none';
// On every 'Change' of the drop down with the ID "FavoriteColorDropDown" call the displayTextField function
document.getElementById("FavoriteColorDropDown").addEventListener("change", displayTextField);
function displayTextField() {
// Get the value of the selected drop down
var dropDownText = document.getElementById("FavoriteColorDropDown").value;
// If selected text matches 'Other', display the text field.
if (dropDownText == "Other") {
document.getElementById("EnterFavoriteColorLabel").style.display = 'block';
} else {
document.getElementById("EnterFavoriteColorLabel").style.display = 'none';
}
}
</script>
Add the script after the form elements. The Contact Form 7 form editor should look like this.
Remember, no blank lines in the inline JavaScript or it won’t work.
Conditional Fields In Action – Drop Down
Here is the result of our inline js.
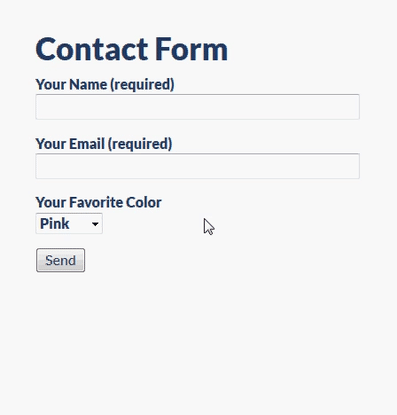
Conditionally Fields In Contact Form 7
Conditionally Display Text Field When A Radio Button Group Selection Is “Other”
For radio buttons, we get the value of the checked radio and when the value is “Other”, we can display the text field.
In this example, we will look at radio buttons that ask for your shirt size with an “Other” option. Just in case if you are an alien.
The inline comments will explain the code.
<label> Your Name (required)
[text* your-name] </label>
<label> Your Email (required)
[email* your-email] </label>
<label>Select A Size:</label> [radio select-a-size id:SelectASizeRadio default:1 "S" "M" "XL" "Other"]
<label id="EnterYourSize"> Please Specify Your Size
[text size] </label>
[submit "Send"]
<script language="javascript" type="text/javascript">
// Hide the Text field by default
document.getElementById('EnterYourSize').style.display = 'none';
document.getElementById('SelectASizeRadio').addEventListener('click', displayTextField);
function displayTextField() {
// Get the value of the currently selected radio button. 'select-a-size' is the name of the radio buttons you specify in the form builder
var radioText = document.querySelector('input[name="select-a-size"]:checked').value;
if (radioText == 'Other') {
document.getElementById('EnterYourSize').style.display = 'block';
} else {
document.getElementById('EnterYourSize').style.display = 'none';
}
}
</script>
Conditional Fields In Action – Radio Buttons
Here is how the inline js works with the radio buttons.
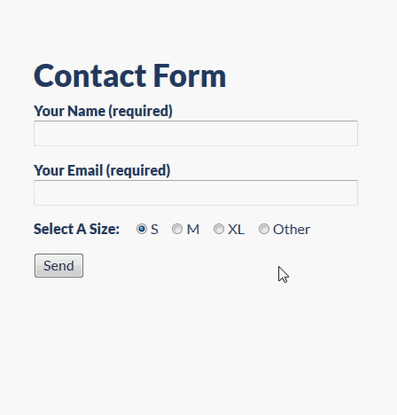
Conditional Text Field With Radio Buttons
No add-on plugins. Just simple plain old JavaScript.
Hope this helps. Feel free to ask for help if you need some hand-holding.
Hi,
Thanks for the javascript code. It works great for our single answer questions!
I was wondering if you maybe can help me with a problem. I’m working on a contact form right now. Do you know if there is a conditional fields code for showing text when all checkboxes are selected? We want to show a message but not until all the checkboxes are selected.
The question people must answer is:
What do you think is the greatest risk of getting sick?
0 Purchase of animals
0 Livestock farmers in the area
0 Animals in nature areas
Answer (shown when all the checkboxes are selected):
All answers are good. The purchase of animals is the greatest risk, but also in other ways, viruses can enter the livestock.
Hope to hear from you soon.
Kind regards.
Hey Sanne,
Here is your answer: https://millionclues.com/wordpress-tips/show-field-all-checkboxes-checked-contact-form-7/
Please let me know if that helps 🙂
Did this help Sanne?
You are amazing. I dont know anything about coding but I just followed this article for inserting multiple conditional fields in the form of my website.
It worked! My first javascript!!!!
Also, my usage was prompted by the opposite of your intro. I have multiple conditional logics and the plugin you talked about becomes very slow when there are more than 50 of them. I have thousands! Using excel now to generate code snippets for the code lol.
Works great – but only for one instance. I have 3 conditional dropdowns on the same form, and only the first one works?
Hi
I have used your answer to solve my problem. it is working. but when the user enters the text . he is not able to click on any other field.
Please help
Hey Aamna, was the user able to enter text before adding the js?
Hi – Your tutorial has been most helpful. One question, how can I get the javascript to work if I have two conditional items in one question (there are four choices total, two of which have the requirement for additional information). I want the user to fill out an employee name if company representative is selected and to provide a name if other is selected. Thanks in advance for your help!
Was able to figure this out – javascript wasn’t filled out correctly 🙂
Thanks for reporting back Cory. Glad you were able to figure it out 🙂
Hey Cory – could you please share how you figured this out? 🙂
Hello Arun nice tutorial can you maybe help me out with something im looking for the next
I want a radiobutton with 3 or 4 options
if an option is selected i want to make drop down with time options visible
so radiobuttons with options like:
0 appointment 1
0 appointment 2
0 appointment 3
0 appointment 4
if radio 1 or 2 is selected i want to make dropdown Time A visible
time A
[select tijden multiple “10:00” “11:45” “13:30”]
if radiobutton 3 or 4 is selected i want to make dropdown time B visible
time B
[select tijden multiple “17:00” “18:45” “20:30” “22:15”]
Hope you can help me out with that one thnx in advanced,
Joop
Hey Joop, I honestly hope that you figure it out. While I would like to help you out, I do not currently have the free time to figure it out for you. Sorry about that.
Good luck!
Hello Arun Basil Lal,
I am facing one issues. When we select “Other” select options and fill up other options text field and again select “Red” color. Then “Other” options filled up text field is hide. But in email body mail I have received that other options text field value also.
So my question is that if use first select “Other” option and then select “Red” options then how clear that “Other” options text field values. So in mail body i can’t receive that value.
Thanks.
Hey Ketan,
Replace the else block in the javascript with:
document.getElementById('EnterYourSize').style.display = 'none';
document.getElementById('EnterYourSize').value = '';
Hope that makes sense.
Hi Arun,
I found this code VERY useful, but I have a question: I have a form with the option to add the mobile number, this field is required. So when the user turns the radio button into YES, the form will show this field to fill. If the user turns the option into NO, the field will collapse, BUT I need to put a “000” value into it, so the form will not show empty field error when submit.
I tried to put a default value into the field, but this doesn’t work because users forget to add their mobile number and the form comes to me with this field empty…
Any help or reference will be much appreciated!
Thank you
Hello Alejandra, Thanks for the kind words.
Why not make the text-box a non-mandatory field (while still saying it is mandatory)? That way there won’t be a validation error.
Conditional Fields In Action – Drop Down Doesn’t Work, but Radio Buttons is Work Properly. Help my problem..
Thanks for the post, I have somewhat different scenario, based on select input i need to create no. of input fields, so if user select 2 in drop down then 2 input fields should display , how can we achieve that ?
Also, how you handled the email sending part, because we need to set values there untill and unless we have some values in input ?
Works great – but only for one instance. I have 3 conditional dropdowns on the same form, and only the first one works?
Hi, I have 2 radio buttons in my contact form. and I want to show different field click on each button. Can you help me? I am using Contact form 7 plugin.
Hi,
I need to make the displayed text field when option ‘Other’ is selected be required, but no when it is not selected. If not, the form keeps asking there is one error but the user doen’t see it because didn’t select the ‘Other’ option.
How do I do that?
Thank you so much. for the code. It saved my time
Hi, great tutorial you have here, how about changing the none required field to make it required using the same drop down?
Hi Arun,
I’m hoping you can help! I have a cf7 form dropdown field which I want to populate from values in a simple html form in a product description in Woocommerce which allows users to select a date which then sends with the form. Can you please help? I cannot find anything anywhere that helps.
Many thanks!!
I have 3 conditional dropdowns for example if I select the option country like India then the next dropdown should populate the states in India and for other countries also it will repeat the same. If I have 10 states and the user selects the option Other then the input field has to populate. This should work on other conditional dropdowns also.
How can I achieve this?
Thank you in advance
There are add-on plugins that do this I believe https://wordpress.org/plugins/country-state-city-auto-dropdown/
i select the pink color to the dropdown
and use the text* require field in other select option to the text field form display error
One or more fields have an error. Please check and try again.